Swap two, three of more values in JavaScript
Best and easiest way to swap two, three of more values in JavaScript.
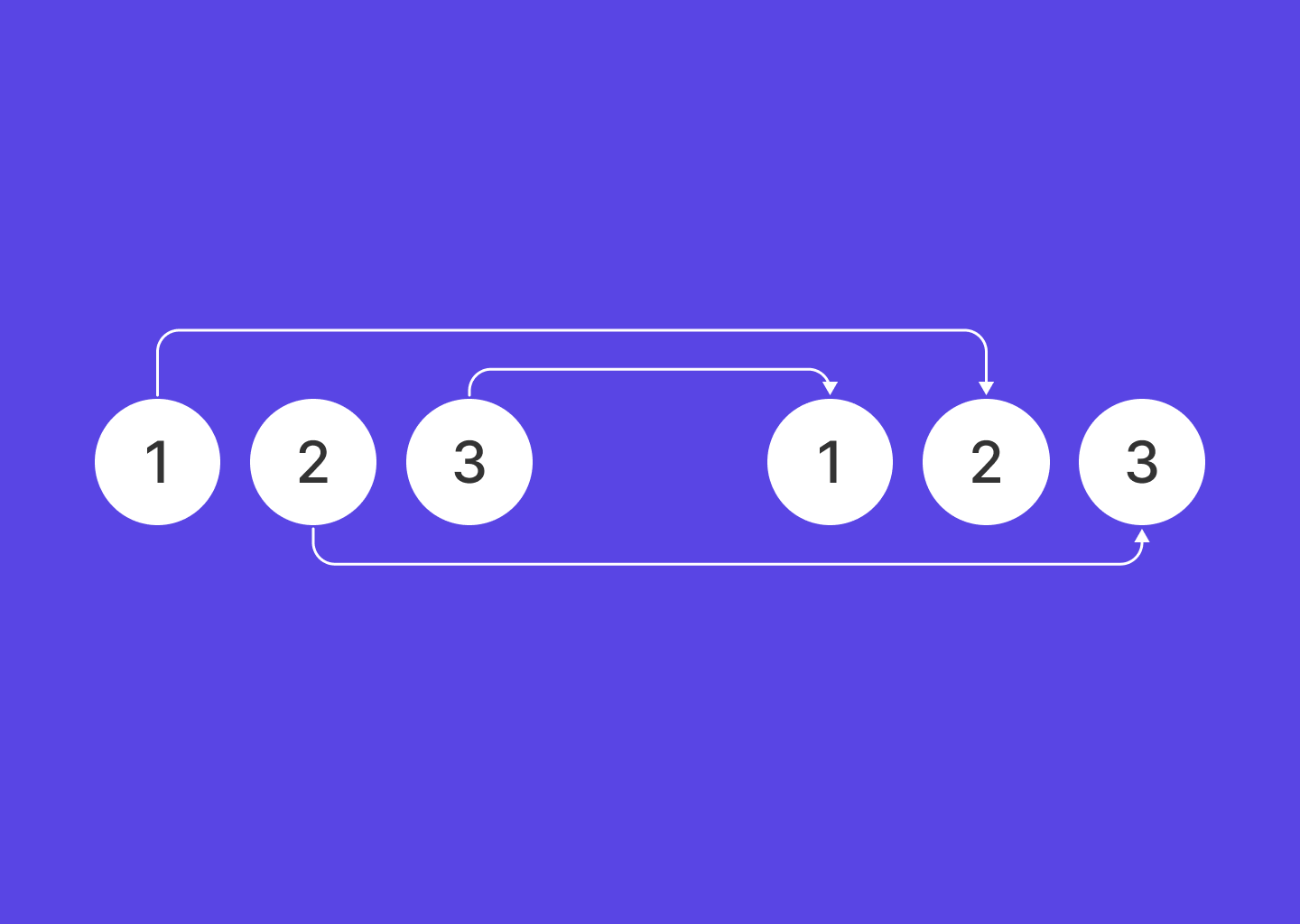
Published At
5/4/2021
Reading Time
~ 2 min read
Here is the way:
let [a, b, c] = [10, 20, 30]
;[a, b, c] = [b, c, a]
// swapped: a = 20, b = 30, c = 10
let [d, e] = [40, 50]
;[d, e] = [e, d]
// swapped: d = 50, e = 40
let [a, b, c] = [10, 20, 30]
;[a, b, c] = [b, c, a]
// swapped: a = 20, b = 30, c = 10
let [d, e] = [40, 50]
;[d, e] = [e, d]
// swapped: d = 50, e = 40
Now lets jump into details. For swapping, traditionally I used to do with temporary variable, like:
let a = 10,
b = 20
let temp = a
a = b
b = temp
// a = 20, b = 10
let a = 10,
b = 20
let temp = a
a = b
b = temp
// a = 20, b = 10
But there was a better way to same in python:
a = 10
b = 20
a, b = b, a
# a = 20, b = 10
a = 10
b = 20
a, b = b, a
# a = 20, b = 10
and then come the es6 with the array destructuring, like:
let [a, b] = [10, 20]
let [c, d, e, ...rest] = [30, 40, 50, 60, 70, 80]
// a = 10, b = 20, c = 30, d = 40, e = 50, rest = [60, 70, 80]
let [a, b] = [10, 20]
let [c, d, e, ...rest] = [30, 40, 50, 60, 70, 80]
// a = 10, b = 20, c = 30, d = 40, e = 50, rest = [60, 70, 80]
so, we can use the array destructuring to swap the value.
Here is a bubble sort
function to understand it better:
function bubbleSort(array) {
let isSorted = false
while (!isSorted) {
isSorted = true
for (let i = 0; i < array.length - 1; i++) {
if (array[i] > array[i + 1]) {
isSorted = false
[array[i], array[i + 1]] = [array[i + 1], array[i]]
}
}
}
return array
}
function bubbleSort(array) {
let isSorted = false
while (!isSorted) {
isSorted = true
for (let i = 0; i < array.length - 1; i++) {
if (array[i] > array[i + 1]) {
isSorted = false
[array[i], array[i + 1]] = [array[i + 1], array[i]]
}
}
}
return array
}
🙏
Do you have any questions, or simply wish to contact me privately? Don't hesitate to shoot me a DM on Twitter.
Have a wonderful day.
Abhishek 🙏