Create an Animated Signature
Learn to Craft an Animated Signature Using SVG, React, and Framer Motion
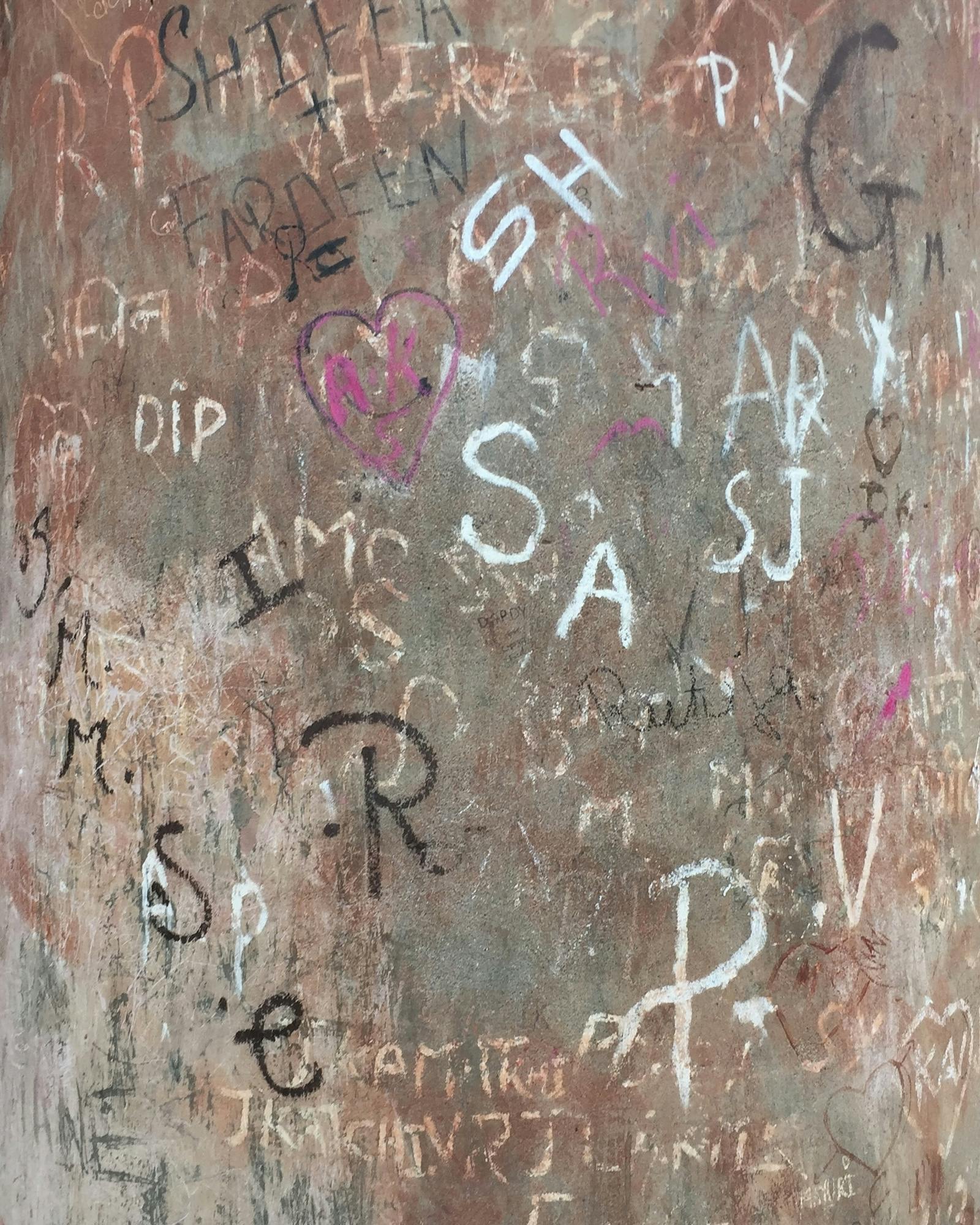
Published At
12/20/2023
Reading Time
~ 7 min read
Introduction
In the digital age, personalization is crucial for standing out. And what better way to add a personal touch to your website or online portfolio than with an animated signature? An animated signature not only showcases your creativity but also adds sophistication to your digital presence.
This tutorial will guide you through the process of creating an animated signature using SVG (Scalable Vector Graphics), React, and the Framer Motion library. We'll begin by designing the signature using Figma on an iPad, taking advantage of the ease of a pen over a mouse. Then, we'll explore the technical aspects of bringing this signature to life using React and Framer Motion, a powerful library that simplifies animations in React and makes them accessible.
Designing the Signature
Choosing the Right Tools
The first step in creating an animated signature is designing the signature itself. For this tutorial, we will use Figma, a popular digital design tool. Figma's intuitive interface and compatibility with touch devices like the iPad make it an excellent choice for this task. Additionally, using an iPad and a pencil (or your finger) allows for a more natural drawing experience, closely mimicking the act of signing on paper. However, you can still accomplish this with a mouse on a laptop.
Crafting Your Signature
- Open Figma and create a new project.
- To draw your signature, use the pencil tool. Don't worry if it's not perfect on the first try (it took me around 10 to 12 tries). Figma allows you to edit the paths as needed.
- Once satisfied, export your signature as an SVG file. SVG is ideal for web use, as it maintains quality at any scale and is easily animatable.
Setting Up Your React Project
Initial Setup
Before diving into the code, make sure you have a React environment set up. If you're starting from scratch, create a new React app using create-react-app
or nextjs
.
- Go to your project directory and install the required dependencies, including Framer Motion, by executing the following command:
npm i framer-motion
npm i framer-motion
Introduction to Framer Motion
Framer Motion is a powerful library for creating animations in React applications. It provides a set of components that extend standard HTML and SVG elements, making it easy to animate elements with minimal code.
Key Concepts
- Motion Components: Framer Motion extends standard components like
div
,svg
, andpath
tomotion.div
,motion.svg
, andmotion.path
, enabling animations. - Variants: These are sets of visual states that your component can animate between, such as
hidden
andvisible
. - Animate Presence: This component allows for more complex animations.
Develop the Animated Signature Component
Let's dive into the code for our animated signature.
Setting Up the Component
- To begin, create a new component called
AnimatedSignature.tsx
in your project. - Import React, useRef, and the necessary Framer Motion components.
import React, { useRef } from 'react';
import { motion, useInView } from 'framer-motion';
import React, { useRef } from 'react';
import { motion, useInView } from 'framer-motion';
Using useRef and useInView
- The
useRef
hook in React allows us to reference elements in our component. - The
useInView
hook in Framer Motion detects when an element is visible in the viewport. It can be used with theanimate
property inmotion.svg
, as we will see later in the blog, to trigger the animation only when the element is in view.
const ref = useRef(null);
const isVisible = useInView(ref);
const ref = useRef(null);
const isVisible = useInView(ref);
Defining the Animation Variants
- We will define two variants:
hidden
andvisible
. - The
hidden
variant will set the initial state of the signature with zero opacity and path length. - The
visible
variant will define the end state with full opacity and path length.
const draw = {
hidden: {
opacity: 0,
pathLength: 0,
},
visible: {
opacity: 1,
pathLength: 1,
transition: {
pathLength: {
delay: 0.2,
type: 'spring',
duration: 2.5,
bounce: 0.2,
ease: 'easeInOut',
},
opacity: { duration: 2 },
},
},
};
const draw = {
hidden: {
opacity: 0,
pathLength: 0,
},
visible: {
opacity: 1,
pathLength: 1,
transition: {
pathLength: {
delay: 0.2,
type: 'spring',
duration: 2.5,
bounce: 0.2,
ease: 'easeInOut',
},
opacity: { duration: 2 },
},
},
};
Implementing the Signature SVG
- Now, paste your SVG signature under the
return
statement.
return (
<div>
<svg width="1196" height="655" viewBox="0 0 1196 655" fill="none" xmlns="http://www.w3.org/2000/svg">
<path d="M26.8458 57...849 1116.78 75.4613" stroke="black" stroke-width="16" stroke-linecap="round"/>
<path d="M661.928 92...3968 649.055 92.6256" stroke="black" stroke-width="16" stroke-linecap="round"/>
<path d="M121.25 646....503.617C548.496 448.539 677.727 386.9 811.401 335.549C900.688 301.25 998.458 278.787 1078.16 225.649" stroke="black" stroke-width="16" stroke-linecap="round"/>
<path d="M1176.86 1...171.976 1149.96 195.612" stroke="black" stroke-width="16" stroke-linecap="round"/>
</svg>
</div>
)
return (
<div>
<svg width="1196" height="655" viewBox="0 0 1196 655" fill="none" xmlns="http://www.w3.org/2000/svg">
<path d="M26.8458 57...849 1116.78 75.4613" stroke="black" stroke-width="16" stroke-linecap="round"/>
<path d="M661.928 92...3968 649.055 92.6256" stroke="black" stroke-width="16" stroke-linecap="round"/>
<path d="M121.25 646....503.617C548.496 448.539 677.727 386.9 811.401 335.549C900.688 301.25 998.458 278.787 1078.16 225.649" stroke="black" stroke-width="16" stroke-linecap="round"/>
<path d="M1176.86 1...171.976 1149.96 195.612" stroke="black" stroke-width="16" stroke-linecap="round"/>
</svg>
</div>
)
- Remove any unwanted code from it.
- Convert
div
,svg
,path
, tomotion.div
,motion.svg
,motion.path
. - Apply the
draw
variants which we created above, to themotion.path
elements. - Use the
initial
,animate
, andref
props to control the animation.
return (
<motion.div ref={ref}>
<motion.svg
// SVG properties
initial="hidden"
animate={isVisible ? 'visible' : 'initial'}
>
<motion.path
// Path properties
variants={draw}
/>
{/* Include other paths if your signature has multiple parts */}
</motion.svg>
</motion.div>
);
return (
<motion.div ref={ref}>
<motion.svg
// SVG properties
initial="hidden"
animate={isVisible ? 'visible' : 'initial'}
>
<motion.path
// Path properties
variants={draw}
/>
{/* Include other paths if your signature has multiple parts */}
</motion.svg>
</motion.div>
);
Animation Properties and Customization
The draw
variants control how the signature animates. You can customize these to change the animation's look and feel.
- Opacity: Controls the visibility of the signature.
- PathLength: Determines the drawing effect of the signature.
- Transition: Defines the timing and type of the animation.
Experiment with different values for delay
, duration
, bounce
, and ease
to achieve various effects.
Integrating the Component into a Web Page
Now that our AnimatedSignature
component is ready, integrating it into a web page is straightforward.
- Import
AnimatedSignature
into your desired React component or page. - Insert
<AnimatedSignature />
where you want the signature to appear. - Style and position the signature as needed, considering responsiveness and layout.
Conclusion
Animated signatures are a unique way to add personality and elegance to your web projects. By following this guide, you now have a beautiful, animated signature in your React application. Don't be afraid to experiment with different animations and styles. The world of web animation is vast, and Framer Motion makes it accessible and fun.
Remember, this is just the beginning. You can apply these principles to animate other SVGs and UI elements, unlocking endless possibilities for interactive and dynamic web experiences. Happy coding!
Do you have any questions, or simply wish to contact me privately? Don't hesitate to shoot me a DM on Twitter.
Have a wonderful day.
Abhishek 🙏