Palindrome Check
Grasp the concept of palindromes in programming and learn to check strings efficiently for this symmetry.
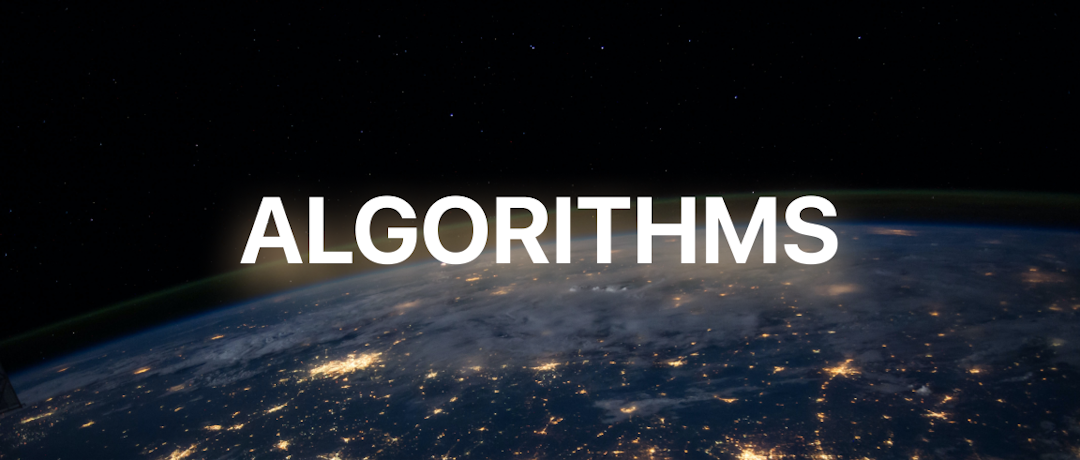
Published At
6/4/2021
Reading Time
~ 2 min read
Write a function that takes in a non-empty string and that returns a boolean representing whether the string is a palindrome.
A palindrome is defined as a string that's written the same forward and backward. Note that single-character strings are palindromes.
Sample Input
string = "abcdcba"
string = "abcdcba"
Sample Output
true // it's written the same forward and backward
true // it's written the same forward and backward
Hints
Hint 1
Start by building the input string in reverse order and comparing this newly built string to the input string. Can you do this without using string concatenations?
Hint 2
Can you optimize your algorithm by using recursion? What are the implications of recursion on an algorithm's space-time complexity analysis?
Hint 3
Go back to an iterative solution and try using pointers to solve this problem: start with a pointer at the first index of the string and a pointer at the final index of the string. What can you do from there?
Optimal Space & Time Complexity
O(n) time | O(1) space - where n is the length of the input string
function isPalindrome(string) {
if (string.length === 1) return true;
if (string.length === 2) {
if (string[0] === string[1]) {
return true
} else return false
}
string.charAt[string.length]
if (string[0] === string[string.length - 1]) {
let sliced = string.slice(1, string.length - 1)
return isPalindrome(sliced);
}
return false
}
function isPalindrome(string) {
if (string.length === 1) return true;
if (string.length === 2) {
if (string[0] === string[1]) {
return true
} else return false
}
string.charAt[string.length]
if (string[0] === string[string.length - 1]) {
let sliced = string.slice(1, string.length - 1)
return isPalindrome(sliced);
}
return false
}
function isPalindrome(string) {
let reversedString = '';
for (let i = string.length - 1; i >= 0; i--) {
reversedString += string[i];
}
return string === reversedString;
}
function isPalindrome(string) {
let reversedString = '';
for (let i = string.length - 1; i >= 0; i--) {
reversedString += string[i];
}
return string === reversedString;
}
🐉
Do you have any questions, or simply wish to contact me privately? Don't hesitate to shoot me a DM on Twitter.
Have a wonderful day.
Abhishek 🙏